In Flutter, field validation is as simple as “hello world”. You do not need a 3rd party package to get this done, because flutter takes off the tedious work from your neck.
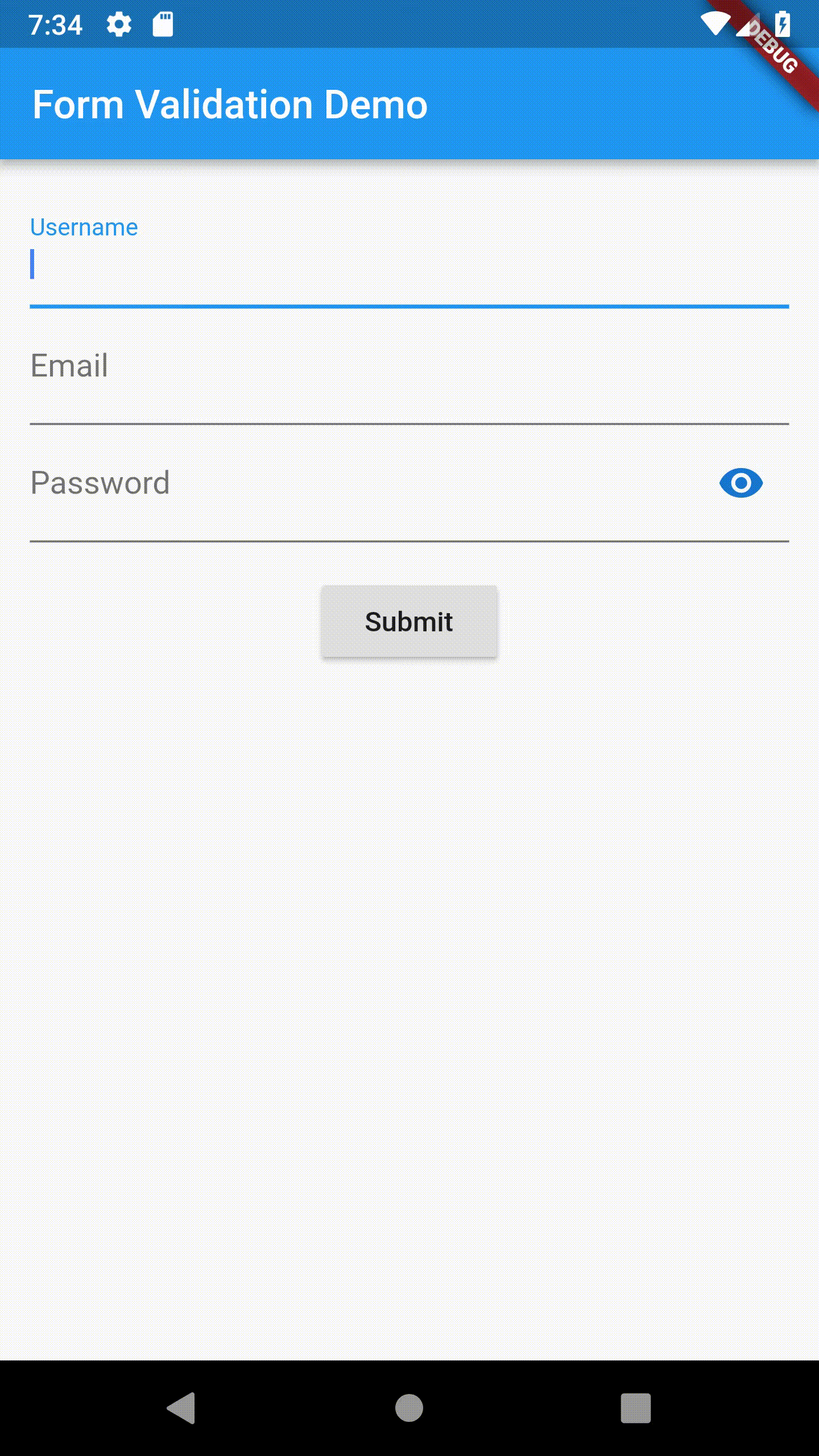
In this article, we will see how you can validate regular form fields as follows:
- Name
- Password
Name Validation
Step 1:
In flutter, we can validate an input field by passing a custom validation function to TextFormField’s validator property. So let’s create a widget with one TextFormField .
// Build a custom widget Widget formUI() { return new Column( crossAxisAlignment: CrossAxisAlignment.center, children: <Widget>[ new TextFormField( decoration: const InputDecoration(labelText: 'Name'), // Create an optional decoration for your TextFormField validator: _validateName, //We will create this function in step 2 ), .... ], ); }
Step 2:
Create a function called _validateName to complete the code snippet in step 1.
_validateName(String value){ if(value.isEmpty){ return 'Name cannot be empty'; }
Email Validation
Step 1:
Add a new TextFormField to the formUI we created under step 1 of Name validation.
// Build a custom widget Widget formUI() { return new Column( crossAxisAlignment: CrossAxisAlignment.center, children: <Widget>[ new TextFormField( decoration: const InputDecoration(labelText: 'Name'), // Create an optional decoration for your TextFormField validator: _validateName, //We will create this function in step 2 ), new TextFormField( decoration: const InputDecoration(labelText: 'Email'), validator: _validateEmail, keyboardType: TextInputType.emailAddress, ), ], ); }
Step 2:
Create a function called _validateEmail to complete the code snippet in step 1.
_validateEmail(String value) { if (value.isEmpty) { return 'Email field cannot be empty!'; } // Regex for email validation String p = "[a-zA-Z0-9\+\.\_\%\-\+]{1,256}" + "\\@" + "[a-zA-Z0-9][a-zA-Z0-9\\-]{0,64}" + "(" + "\\." + "[a-zA-Z0-9][a-zA-Z0-9\\-]{0,25}" + ")+"; RegExp regExp = new RegExp(p); if (regExp.hasMatch(value)) { return null; } return 'Email provided isn\'t valid.Try another email address'; }
Password Validation
Step 1:
Add a new TextFormField to the formUI we created under step 1 of Email validation.
// Build a custom widget Widget formUI() { return new Column( crossAxisAlignment: CrossAxisAlignment.center, children: <Widget>[ new TextFormField( decoration: const InputDecoration(labelText: 'Name'), // Create an optional decoration for your TextFormField validator: _validateName, //We will create this function in step 2 ), new TextFormField( decoration: const InputDecoration(labelText: 'Email'), validator: _validateEmail, keyboardType: TextInputType.emailAddress, ),
new TextFormField( keyboardType: TextInputType.text, validator: _validatePassword, decoration: const InputDecoration( labelText: 'Password', hintText: 'Enter your password', ), ), ], ); }
Step 2:
Create a function called _validatePassword to complete the code snippet in step 1.
_validatePassword(String value){ if(value.isEmpty){ return 'Password field cannot be empty'; } // Use any password length of your choice here if(value.length<6){ return 'Password length must be greater than 6'; } }
To quickly get started with Flutter, you can use the following links.
Official Flutter Documentation
Flutter Cheat Sheet for beginners
Do you love what you just read? Kindly share with someone.
No Comment! Be the first one.