
It’s amazing to see how much time and resources the Kotlin team has invested in developing some great and helpful tools to make Android development easier. In this article, I’ll show you how Anko library can make your life better :). In addition, I will share different ways you can use Anko in your android development.
What is Anko?
Anko is a very robust library developed by JetBrains. Its main purpose is the generation of UI layouts by using code instead of XML. This is a fascinating feature I recommend you to try out in your next app development.
Is this the only feature we can get from this library?
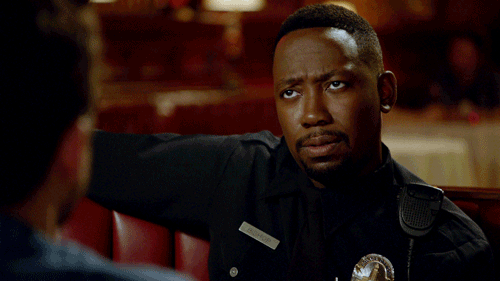
Absolutely not!!!
Anko includes a plethora of extremely helpful functions and properties that will avoid lots of boilerplate. We will see several use cases throughout this articles, but you’ll quickly see which kind of problems this library solves.
Since Anko is a library written specifically for Android, I recommend you to understand what it is doing behind the scenes. You can navigate at any moment to Anko source code using ctrl + click (Windows or Linux) or cmd + click (Mac). Anko implementation is an excellent example to learn useful ways to get the most out of Kotlin language.
Before going any further, let’s use Anko to simplify some code. As you will see, anytime you use something from Anko, it will include an import with the name of the property or function to the file. This is because Anko uses extension functions to add new features to Android framework. We’ll take a look at what an extension function is and how to write one.
Take for instance, In ListActivity:onCreate, an Anko extension function can be used to simplify how to find the RecyclerView:
val todoList: RecyclerView = find(R.id.todo_list)
Alert Dialogs
Anko provides a declarative way of creating alert dialogs with text messages, lists, progress bars and even with your own DSL layout.
Take for instance, If you want to display an alert message to users with a couple of buttons at the bottom, all you need is:
alert("Contact", "Do you want to call Dad?") { positiveButton("Yes") { processAnOrder() } negativeButton("No") { } }.show()
There is a function that creates and shows a list dialog:
val countries = listOf("Nigeria", "France", "United States") selector("What is your favorite country?", countries) { i -> toast("So your country is ${countries[i]}, right?") }
Both indeterminate and basic progress dialogs are supported:
progressDialog("Please wait a minute.", "Downloading…") indeterminateProgressDialog("Fetching the data…")
I mentioned initially that you can create your own DSL layout in dialogs. This is how to create a custom layout:
alert { customView { verticalLayout { val familyName = editText { hint = "Family name" } val firstName = editText { hint = "First name" } positiveButton("Register") { register(familyName.text, firstName.text) } } } }.show()
Intent Callers
In Android, every application has code that loads a page in the default web browser or launches a new email activity using intents, so there are helper functions for this in Anko:
browse("http://somewebsite.org (http://somewebsite.org/)")
email("[email protected] ([email protected])", "Here I am!", "Hello World")
Services
Android system services, such as WifiManager
, LocationManager
orVibrator
, are available in Anko through extension properties for the Context
:
if (!wifiManager.isWifiEnabled()) { vibrator.vibrate(200) toast("Wifi is disabled. Please turn on!") }
Intent
The common way of starting a new Activity
is to create an Intent
, maybe put some parameters into it, and finally pass the created Intent
to the startActivity()
method of a Context
.
val intent = Intent(this, javaClass<SomeActivity>()) intent.putExtra("id", 5) intent.putExtra("firstName", "Temidayo") startActivity(intent)
With Anko we can do this in exactly one line of code:
startActivity<RandomActivity>("id" to 1, "userName" to "Temidayo")
startActivity()
function accepts key-value pairs that will be passed as Intent
extra parameters. Another function, startActivityForResult()
with similar semantics is also available.
Setup Anko in your project
Finally,
Adding Anko to your project is quiet easy. You can add the following dependencies:
ankoVersion = "0.10.1" dependencies { compile "org.jetbrains.anko:anko-appcompat-v7-listeners:$ankoVersion" compile "org.jetbrains.anko:anko-design-listeners:$ankoVersion" compile "org.jetbrains.anko:anko-design:$ankoVersion" compile "org.jetbrains.anko:anko-sdk15-listeners:$ankoVersion" compile "org.jetbrains.anko:anko-sdk15:$ankoVersion" }
You are all set!!!
No Comment! Be the first one.