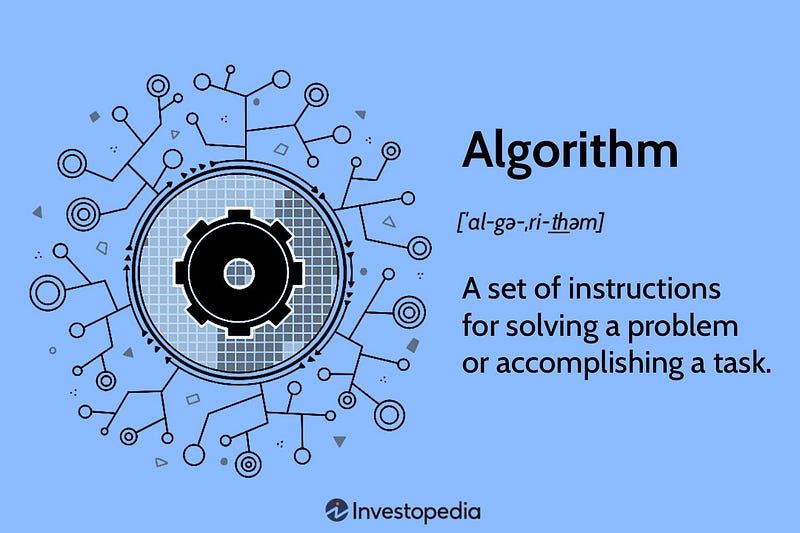
If you’re new to software development and want to grasp the basics of computer science, you’ve come to the right place. In this cheat sheet, I’ll walk you through the fundamental concepts of computer science in a concise and easy-to-understand manner. Let’s dive in!
Hardware Fundamentals
Central Processing Unit (CPU)
– The CPU is like the brain of a computer. It takes data from the main memory, processes it using its subunits, and then returns the updated data to the main memory.
– The Control Unit (CU) is a vital part of the CPU responsible for controlling the flow of data between the CPU and the main memory.
– The Arithmetic and Logic Unit (ALU) is another crucial component of the CPU that handles arithmetic operations (like addition and subtraction) and logic operations (like comparisons and Boolean operations).
Input Units
– Input units are the computer’s way of receiving data from the outside world or input devices. They take in this data and convert it into streams of bytes that the computer can understand.
– Common input devices include keyboards, mice, scanners, and various sensors.
Output Units
– Output units play the opposite role of input units. They take processed data from the CPU and present it in a format that humans can comprehend.
– Examples of output devices include monitors, printers, speakers, and actuators.
Storage Units
– Storage units are where data is stored after being retrieved and processed by the computer.
– The main memory, including Random Access Memory (RAM), refers to the physical spaces in the computer where data and instructions are temporarily stored while the computer is running.
– Secondary storage devices, such as hard drives and solid-state drives, provide long-term storage for files and programs.
Software Fundamentals
Machine Language
– Machine language is the only language that a computer can directly understand, consisting of binary code (sequences of ones and zeros).
– It is a low-level language that represents instructions specific to a particular computer architecture.
Assembly Language
– Assembly language is a human-readable language that translates mnemonic codes (assembly instructions) into machine language.
– Assembly language instructions are specific to a particular computer architecture and must be assembled into machine language before execution.
High-Level Programming Languages
– High-level programming languages allow programmers to write human-readable programs using statements and constructs closer to natural language.
– These languages abstract away the low-level details of the computer’s architecture and provide a more efficient and productive development environment.
Assembler
– An assembler is a utility program that translates assembly language programs into machine language, using a process called assembly.
Compiler
– A compiler is a program that translates human-readable source code written in a high-level programming language into machine-readable target code.
– The target code is typically in a low-level language and is executed directly by the computer.
Interpreter
– An interpreter translates and executes high-level source code line by line while the program is running.
– It converts the source code into machine language instructions on the fly.
Operating System
– An operating system is a software system that manages computer hardware and software resources, provides common services, and supports the execution of other programs.
– It acts as an intermediary between the user and the computer hardware, providing an interface for users to interact with the system.
User Applications
– User applications refer to software programs designed for end-users to carry out specific tasks unrelated to the operation of the computer system.
– Examples include word processors, web browsers, video games, and productivity tools.
Data Structure Fundamentals
Data structures are formats used to organize, manage, and store data efficiently, enabling easy access and modification. Let’s explore some commonly used data structures and their characteristics.
Array
– An array is a collection of items of the same variable type that are stored sequentially in memory.
– Arrays provide constant-time retrieval of data by using an index to access elements directly.
– However, they are not efficient for fast data insertion or deletion, as the elements need to be shifted or moved accordingly.
Linked List
– A linked list is a linear sequence of nodes, where each node contains a value and a pointer to the next node in the list.
– Linked lists excel in fast data insertion and deletion operations since only the affected nodes need to be modified.
– However, data retrieval in linked lists is slower compared to arrays, as elements must be traversed sequentially.
Tree
– A tree is a non-linear data structure often used to represent hierarchical relationships between data elements.
– It consists of nodes connected by edges, where each node can have child nodes.
– Trees are commonly used in file systems, database indexing, and representing hierarchical data.
Stack
– A stack is a linear data structure that follows the Last-In, First-Out (LIFO) principle.
– Imagine a stack of plates: The last plate placed on top is the first one to be taken out.
– Stack operations include push (adding an element to the top) and pop (removing the top element).
Queue
– A queue is a linear data structure that follows the First-In, First-Out (FIFO) principle.
– Think of a line for a roller coaster: The first people in line are the first to leave for the ride.
– Queue operations include enqueue (adding an element to the rear) and dequeue (removing an element from the front).
Graph
– A graph is an abstract notation used to represent connections between pairs of objects.
– It consists of vertices (objects) and edges (connections between vertices).
– Graphs are widely used in network modeling, social networks, and routing algorithms.
Hash Table
– A hash table is a data structure implemented by storing elements in an array and identifying them through a key.
– A hash function takes a key as input and returns an index where the corresponding value is stored.
– Hash tables offer efficient retrieval, insertion, and deletion of elements when the hash function is well-designed.
Heap
– A heap is an advanced tree-based data structure primarily used for sorting and implementing priority queues.
– It satisfies the heap property, which can be either a min heap (the smallest element is at the root) or a max heap (the largest element is at the root).
– Heaps are efficient for maintaining the highest or lowest element at the root and are used in algorithms like heap sort and priority queues.
Algorithm Fundamentals
An algorithm is a series of well-defined instructions that guide a computer in solving a problem. Algorithms are applied to data structures, enabling efficient data manipulation and processing. Let’s explore some essential concepts and techniques related to algorithms.
Complexity and Correctness Concepts
– Asymptotic Time Complexity: An analysis that determines the exact running time of an algorithm as the size of the input grows. It provides insight into performance regardless of the underlying machine. Notations like Big O (upper bound), Big Omega (lower bound), and Big Theta (tight bound) represent the running time.
– Time Complexity of Recursive Algorithms: Recursive algorithms’ running time can be computed using techniques such as the substitution method, Master’s theorem, or recursion tree.
– Asymptotic Space Complexity: An analysis of how much memory an algorithm requires as the input size increases.
– Correctness Proof Techniques: These techniques verify that an algorithm is correct and consistently produces the intended output. The most widely used technique is loop invariant, which relies on mathematical induction to establish correctness.
Design Techniques
– Brute Force: Exhaustively checking all possibilities to find a solution, often inefficient and time-consuming.
– Divide and Conquer: Breaking a problem into smaller subtasks, solving them recursively, and combining the solutions. Examples include merge sort and quicksort.
– Dynamic Programming: Breaking a big problem into smaller overlapping subtasks, solving them, and storing results in memory (memoization) to avoid redundant computations.
– Greedy: Choosing the best available local solution for each subtask, hoping it leads to the best global solution. Optimality is not guaranteed in all cases.
Other Techniques
– Approximation Algorithms: Finding near-optimal solutions when finding the exact optimal solution is impractical or time-consuming.
– Randomized Algorithms: Utilizing randomness to achieve faster or more efficient results in certain scenarios.
– Linear Programming: Solving optimization problems with linear objective functions and linear constraints.
Key Categories
– Sorting and Searching Algorithms: Organizing elements in order or searching for specific elements in data structures. Examples include mergesort, quicksort, bubble sort, selection sort, insertion sort, linear search, and binary search.
– Graph Algorithms: Solving problems related to representing and manipulating graphs, which are abstract notations that depict connections between objects.
– Shortest Path Algorithms: Finding the most efficient path between two points in a graph. Various algorithms exist, and the choice depends on factors such as data type, size, and application requirements.
Congratulations! You’ve reached the end of this beginner’s guide, equipped with a comprehensive computer science cheat sheet to kickstart your exciting journey into the world of technology.
As you embark on your tech journey, keep in mind the importance of practice, experimentation, and problem-solving. Embrace challenges and approach them with a logical and analytical mindset. Develop your coding skills, enhance your problem-solving abilities, and foster a curious and inquisitive nature.
Utilize this cheat sheet as a handy reference to refresh your knowledge and reinforce your understanding whenever needed. Embrace opportunities to apply these concepts in real-world projects, engage in collaborative learning communities, and seek guidance from experienced mentors.
Computer science is a dynamic and ever-evolving field, and staying up-to-date with the latest trends and technologies is crucial. Embrace a growth mindset and remain adaptable as new advancements continue to shape the industry.
Now, it’s time to take the leap and start applying what you’ve learned. Dive into coding, explore projects, and embrace challenges. Remember that perseverance and dedication are key to mastering software development.
Good luck, and may your tech journey be filled with boundless knowledge, thrilling discoveries, and endless possibilities!
No Comment! Be the first one.