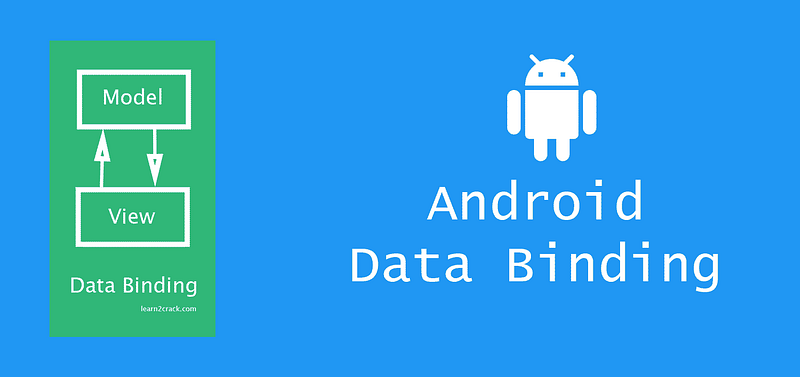
Hello World!!! I really do hope you learnt the entire concept of data binding in my previous article.If you haven’t done so, kindly take a peek at my previous article on how to empower your UI with Android Jetpack.
Today, let’s dive into more interesting part of this component.
There was a time I needed to write a logic to display a TextView if the age of a person is < 12. I needed to go through the conventional way which is to hide and show the TextView in an IF ELSE condition. What if data binding is the magic you need?
Take a look at this…
android:text="@{String.valueOf(index + 1)}"
android:visibility="@{userAge < 12 ? View.GONE : View.VISIBLE}"
android:transitionName='@{"image_" + id}'
With Expression language in data binding, you can combine several simple and complex operators to perform desired operations.
Null coalescing operator
The null coalescing operator (??
) selects the left operand if it isn’t null
or the right if the former is null
.
android:text="@{person.displayName ?? person.firstName}"
The above code is functionally the same as this:
android:text="@{person.displayName != null ? person.displayName : person.firstName}"
Property Reference
Amazingly, an expression can reference a property in a class by using the following format, which is equivalence to fields, getters, and ObservableField
objects:
android:text="@{person.userAge}"
Event handling
Data binding provides you with expression handling events that are dispatched from the views in real time(for instance, the onClick()
method). Event attribute names are determined by the name of the listener method with a few exceptions. A good example is View.OnClickListener
which has a method onClick()
, so the attribute for this event is android:onClick
.
- SearchViewsetOnSearchClickListener(View.OnClickListener)
android:onSearchClick
- ZoomControlssetOnZoomInClickListener(View.OnClickListener)
android:onZoomIn
Let’s say you need to assign an event to its handler, you can use a normal binding expression, with the value being the method name to call. Take a look at the example below:
In your java class
public class MyHandlers {
public void onClickPerson(View view) { ... }
}
In your layout
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android">
<data>
<variable name="handlers" type="com.example.MyHandlers"/>
<variable name="person" type="com.example.Person"/>
</data>
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@{person.lastName}"
android:onClick="@{handlers::onClickFriend}"/>
</LinearLayout>
</layout>
Listener bindings
Listener bindings are simply binding expressions that run when an event happens. Let’s consider the following SpeakerNote class that has the onSaveClick()
method:
public class
SpeakerNote{ public void onSaveClick(View view,Task task){} }
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android">
<data>
<variable name="task" type="com.android.example.Task" />
<variable name="speakernote" type="com.android.example.SpeakerNote" />
</data>
<LinearLayout android:layout_width="match_parent" android:layout_height="match_parent">
<Button android:layout_width="wrap_content" android:layout_height="wrap_content"
android:onClick="@{(theView) -> speakernote.onSaveClick(theView, task)}" />
</LinearLayout>
</layout>
Imports, variables, and includes
The Data Binding Library provides awesome features such as imports, variables, and includes. Imports make easy to reference classes inside your layout files. Variables allow you to describe a property that can be used in binding expressions. Includes let you reuse complex layouts across your app
Imports
Imports allow you to easily reference classes right inside your layout file, just like in managed code. Zero or more import
elements may be used inside the data
element. The following code sample imports the View
class to the layout file:
<data>
<import type="android.view.View"/>
</data>
Variables
You can use multiple variable
elements inside the data
element. Each variable
element describes a property that may be set on the layout to be used in binding expressions within the layout file. The following example declares the user
, image
, and note
variables:
<data>
<import type="android.graphics.drawable.Drawable"/>
<variable name="user" type="com.example.Person"/>
<variable name="image" type="Drawable"/>
<variable name="note" type="String"/>
</data>
Includes
You may pass variables into an included layout’s binding from the containing layout by using the app namespace and the variable name in an attribute. The following example shows included person variables from the name.xml
and contact.xml
layout files:
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:bind="http://schemas.android.com/apk/res-auto">
<data>
<variable name="person" type="com.example.Person"/>
</data>
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<include layout="@layout/name"
bind:person="@{person}"/>
<include layout="@layout/contact"
bind:person="@{person}"/>
</LinearLayout>
</layout>
Data Binding is a very important component every developer should incorporate in their development.
Why should you do this?
- You never have to write findViewById() again.
- Use a MVVM approach rather than MVC which simplifies and separates the presentation layer from the business logic.
- Listener Bindings made easy
I have more and more to share….
I don’t have an app idea yet, but I would like to try out data binding. How can I do this?
Create a simple Registration App that allow users to provide their basic information after which the app takes them to another activity to display all the data provided.
The rule is:
No findViewById();
No setOnClick….
Try as much as possible to use data binding in your development!!!
Let the game begin…
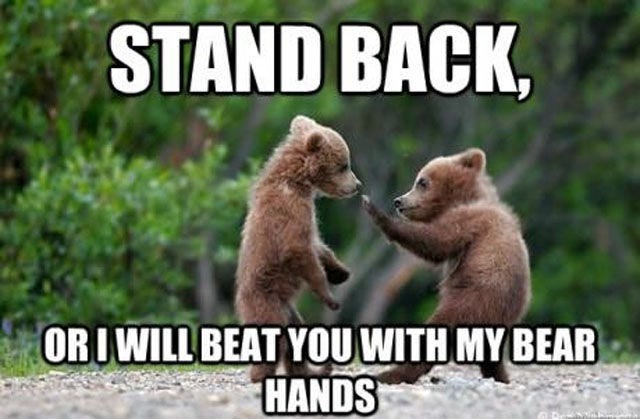
No Comment! Be the first one.