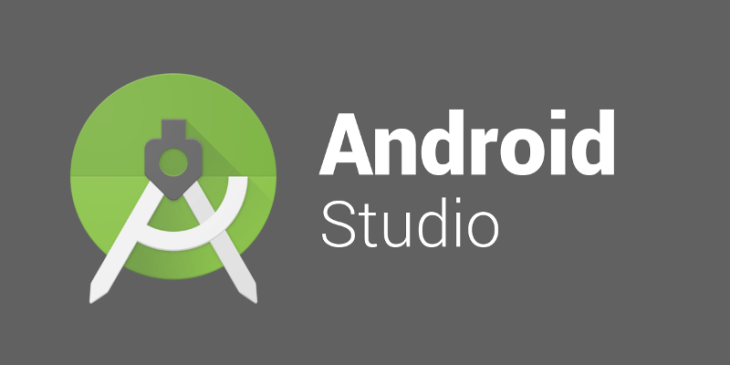
Hello world!!! Welcome to another session of animations using android.In my previous article I discussed extensively how you can use FlingAnimation to create stunning animations in your next android project. If you haven’t read this, I got you!!!. Follow this link to read about it.
Today is quite different as I will sharing tips on how to create awesome effects using Spring physics.
Physics-based motion is driven by force. Spring force, is one such force that guides interactivity and motion. A spring force has the two properties: damping and stiffness. In a spring-based animation, calculation of value and the velocity are dependent on the spring force that are applied on each frame.

If you’d like your app’s animations to slow down in only one direction, consider using a friction-based fling animation instead.
Spring animation life cycle
In a spring-based animation, the SpringForce
class allows you customize final position, its damping ratio, and its spring’s stiffness. As soon as the animation starts, the spring force updates the animation value and the velocity on each frame in real-time. The animation continues until the spring force gets to an equilibrium.
For instance, if you drag an app icon around the screen and later release it by raising your finger from the icon, the icon tugs back to its original place by an invisible but a familiar force.

In order to build a basic spring animation, you can get it done in 3 steps
- Add the support library You must include the support library in your project to use the spring animation classes
- Create a spring animation: The primary step is to create an instance of the
SpringAnimation
class and set the motion behavior parameters. - Start animation: Begin the spring animation.
Optional steps are:
- (Optional) Remove listeners: Remove listeners that are no longer in use.
- Optional) Set a start value: Customize the animation start value.
- (Optional) Set a value range: Set the animation value range to restrain values within the minimum and the maximum range.
- (Optional) Set start velocity: Set the start velocity for the animation.
- (Optional) Set spring properties: Set the damping ratio and the stiffness on the spring.
- (Optional) Create a custom spring: Create a custom spring in case you do not intend to use the default spring or want to use a common spring throughout the animation.
- (Optional) Cancel animation: Cancel the animation in case the user abruptly exits the app or the view becomes invisble.
Take a deep breath and relax!!

Let’s me go further to expound on the points above:
Step 1: Add the support library
To use the physics-based support library, you must add the support library to your project as follows:
- Open the
build.gradle
file for your app module. - Add the support library to the
dependencies
section.
dependencies { implementation 'com.android.support:support-dynamic-animation:27.1.1' }
Step 2: Create a spring animation
The SpringAnimation
class lets you create a spring animation for an object. To build a spring animation, you need to create an instance of the SpringAnimation
class and provide an object, an object’s property that you want to animate, and an optional final spring position where you want the animation to rest.
final View imgView = findViewById(R.id.imageView); // Create a spring animation to animate the view’s translationY property with the final // spring at position 0. final SpringAnimation springAnimator = new SpringAnimation(imgView, DynamicAnimation.TRANSLATION_Y, 0);
The spring-based animation can animate views on the screen by changing the actual properties object’s view. The following views are available in the system:
ALPHA
: Represents the alpha transparency on the view. The value is 1 (opaque) by default, with a value of 0 representing full transparency (not visible).TRANSLATION_X
,TRANSLATION_Y
, andTRANSLATION_Z
: These properties control where the view is located as a delta from its left coordinate, top coordinate, and elevation, which are set by its layout container.TRANSLATION_X
describes the left coordinate.TRANSLATION_Y
describes the top coordinate.TRANSLATION_Z
describes the depth of the view relative to its elevation.ROTATION
,ROTATION_X
, andROTATION_Y
: These properties control the rotation in 2D (rotation
property) and 3D around the pivot point.SCROLL_X
andSCROLL_Y
: These properties indicate the scroll offset of the source left and the top edge in pixels. It also indicates the position in terms how much the page is scrolled.SCALE_X
andSCALE_Y
: These properties control the 2D scaling of a view around its pivot point.X
,Y
, andZ
: These are basic utility properties to describe the final location of the view in its container.X
is a sum of the left value andTRANSLATION_X
.Y
is a sum of the top value andTRANSLATION_Y
.Z
is a sum of the elevation value andTRANSLATION_Z
.
Step 3: Start animation
There are two ways you can start a spring animation: By calling the start()
or by calling theanimateToFinalPosition()
method. Both the methods need to be called on the main thread.
animateToFinalPosition()
method performs two tasks:
- Sets the final position of the spring.
- Starts the animation, if it has not started.
Since the method updates the final position of the spring and starts the animation if needed, you can call this method any time to change the course of an animation. For example, in a chained spring animation, the animation of one view depends on another view. For such an animation, it’s more convenient to use the animateToFinalPosition()
method. By using this method in a chained spring animation, you don’t need to worry if the animation you want to update next is currently running.
The image below illustrates a chained spring animation, where the animation of one view depends on another view.
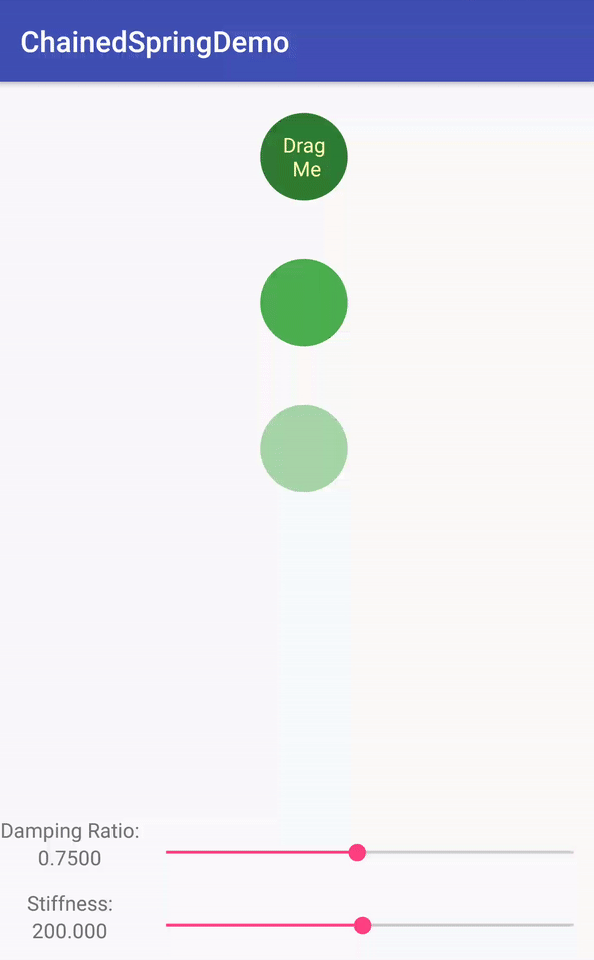
To use the animateToFinalPosition()
method, call the animateToFinalPosition()
method and pass the rest position of the spring. You can also set the rest position of the spring by calling thesetFinalPosition()
method.
The start()
method does not set the property value to the start value immediately. The property value changes at each animation pulse, which happens before the draw pass. As a result, the changes are reflected in the next frame, as if the values are set immediately.
final View imgView = findViewById(R.id.imageView); final SpringAnimation animator = new SpringAnimation(imgView, DynamicAnimation.TRANSLATION_Y); … //Begin the animation animator.start(); …
With 3 basic steps you can animate your view. This is quite easy isn’t it?
Yea, before I wrap up I have a good news for all my readers!!!!
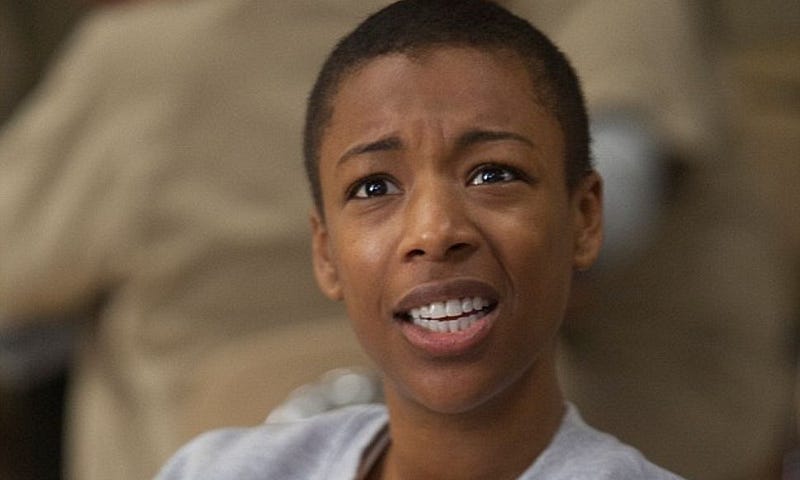
I will be sharing animations created by my readers using Fling and Spring.
Create a minimum of TWO creative using Fling and Spring animations on android studio.
If you would like me to share your creative here, kindly send a mail to [email protected] to indicate interest.
Trust me, in the coming days, my articles are going to be fully packed with a lot of amazing things.
Are you ready for the next baptism???
No Comment! Be the first one.